What is an algorithm and why do we use them in computing?
An algorithm is implemented via a programming language. Although different programming languages have different syntax, similar to French and Italian having different syntax, and may be designed for different purposes, how they implement an algorithm remains roughly the same. They have the same types of structures, although they might work slightly differently or have a different name in different languages. We’re going to look at these structures now to find out more about how they work.
Algorithms are made up of three basic building blocks – sequencing, selection, and iteration.
Sequencing
Sequencing is the order that commands are executed by a computer. A set of logical steps that are carried out in the order we set out to complete a particular task, and if the algorithm has the correct sequence of steps the problem will be solved. If the steps are performed in a different sequence, the result will be different.
We can relate sequence to our everyday life. Think about making a cup of instant coffee as a task. If we want to have a delicious cup of coffee at the end of our task we need to follow the steps in a logical order.
We need to put water in the kettle and switch it on, then get a mug and the coffee from the cupboard, milk from the fridge. We’ll put a spoonful of the coffee and some milk in the mug and can add the steps for sugar if needed. Then we finish with adding the boiling water to the mug as well and stirring it together.
If we did these steps in a different order or left one out, we’d get a very different result! Imagine we wanted to, for example, have a robot make the coffee for us, we’d need to lay out the steps exactly, leaving no detail out.

In programming, computers can only carry out tasks in the sequence they were given to them. They read in a certain order, left to right and top to bottom, and must read code in order. If the sequence of commands is incorrect the computer cannot follow them correctly. It can cause an error in the program we have written, or it may give us an answer we were not expecting. Additionally, if you have an algorithm that produces a result that other algorithms then use to produce further results, if the first result is incorrect, this will have a knock-on effect on the results provided by the later algorithms.
Selection
Selection is a decision or a question in an algorithm, where it reaches a step with several options available. Depending on the answer given to the question, the algorithm will follow certain steps and ignore others. Without selection, we couldn’t include different paths in algorithms, which wouldn’t result in realistic solutions. Imagine we have a simple algorithm to check if you are entitled to an OAP bus fare. The steps would be something like:
- Ask how old you are
- If you are over 65, pay no fare
- Otherwise pay full fare
The decision comes in step 2 where the fare you are charged depends on your age. Selection allows several paths to be included in an algorithm, and selection is usually represented by the instructions IF…THEN…ELSE
- IF represents the question
- THEN points to what to do if the answer to the question is true
- ELSE points to what to do if the answer
Now, if we re-word our algorithm above:
- Ask how old you are
- IF you are over 65 THEN pay no fare
- ELSE pay full fare
When we go through the algorithm with an answer of 66 the answer to the question is true, so the algorithm will tell you to pay no fare. Again, if we repeat with an answer of 64, the answer to the question is false and the algorithm will tell you to pay full fare. You can see that the path followed is dependent on the answer given to the question.

While this is useful, it’s also useful to ‘nest’ if statements together. We can do this using an ELSE IF instruction that allows there to be more than two paths through our algorithm, and any number of ELSE IF instructions can be added to an algorithm. It could look something like:
- IF represents the question
- THEN points to what to do if the answer to the question is true
- ELSE IF represents another question
- THEN points to what to do if the answer to that question is true
- ELSE IF represents another question
- THEN points to what to do if the answer to that question is true
- ELSE points to what to do if the answer to the question is false
Let’s take our bus fare algorithm and extend it out a little more:
- Ask how old you are
- IF you are over 65 THEN pay no fare
- ELSE IF you are under 5 THEN pay no fare
- ELSE IF you are under 16 THEN pay no fare
- ELSE pay full fare
You can see that, depending on the age you give, the ELSE IF statements stop at different points. If you are 67, it stops at point 2, if you are 14 it stops at point 4 and so on. We could make three separate IF statements, although then the 67-year-old would have to check the other IF statements even though they’d already found the correct option. ELSE IF statements are more efficient for your program than multiple IF statements and can be as complex as needed.
Another statement we can use for selection in an algorithm is a CASE statement, sometimes also referred to as a SWITCH statement in some programming languages. It is a type of selection control that allows the flow of program execution to change depending on the answer of a multiway branch and is similar to an ELSE IF statement, but can be more effective in some algorithms depending on the project.
A CASE statement allows us to have as many choices as we want in our selection. Let’s consider an example with four choices:
- If age is equal to 18 you can vote
- Else if age is equal to 39 you’re middle aged
- Else if age is equal to 65 consider retirement
- Else age is unimportant.
The last item is referred to as the default – if your age is not equal to 18, 39 or 65 you get the details message. Let’s see how this would look in JavaScript
Switch(age)
{
Case 18:
Message = “you can vote”;
Break;
Case 39:
Message = “you’re middle aged”;
Break;
Case 65:
Message = “consider retirement”;
Break;
Default:
Message = “age is unimportant”;
Break;
}
The value in the variable age is compared to each ‘case’ in turn using an equality comparison (is age = 18 for example). If it is true, then the message is displayed, and the next line of code (the break) is executed which jumps us to the end of the statement and on to the lines of code after it. If it’s false it moves to the next case and checks it, and so on.
Iteration
Iteration is the process of repeating steps, or a set of steps, over and over a certain number of times, in something called a loop. We’re going to cover the different types of loops later, but all loops will check something called a condition, then either run or not run depending on whether the condition is true. Normally they are designed to run a number of times, for example if i = 0 and we add one each time, run until i = 10 so we repeat the loop 10 times.
The following is a 12-step algorithm for cleaning a person’s upper teeth, and supposes that they have ten top teeth:
- put toothpaste on toothbrush
- use toothbrush to clean tooth 1
- use toothbrush to clean tooth 2
- use toothbrush to clean tooth 3
- use toothbrush to clean tooth 4
- use toothbrush to clean tooth 5
- use toothbrush to clean tooth 6
- use toothbrush to clean tooth 7
- use toothbrush to clean tooth 8
- use toothbrush to clean tooth 9
- use toothbrush to clean tooth 10
- rinse toothbrush
The condition, in this case, will be to check if the number of teeth cleaned equals ten. If that condition is False (the number of teeth cleaned is less than ten), another iteration occurs. When the condition is True (the number of teeth cleaned equals ten), then no more iterations occur.
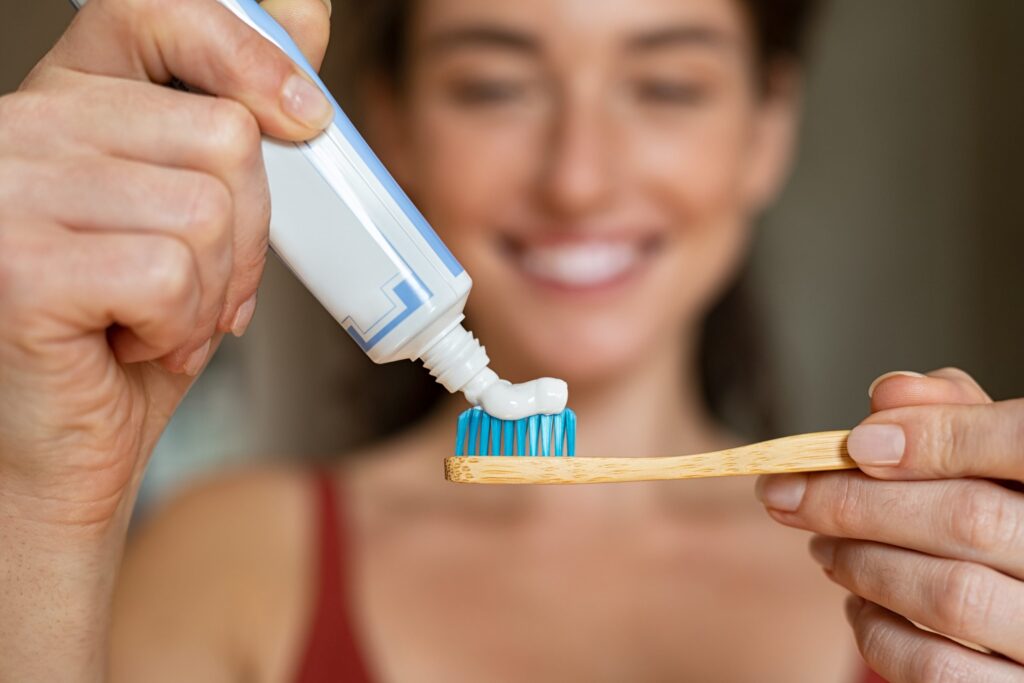
It is also important to keep a count of how many teeth have been cleaned. A counter is used to do this.
The counter can be used to see if the condition has been met. This is known as testing the condition, because the test is whether the condition is True or False.
Now we’ve included a condition and a counter, the algorithm would look like this:
- set number_of_teeth_cleaned to 0
- put toothpaste on toothbrush
- use toothbrush to clean a tooth
- increase number_of_teeth_cleaned by 1
- if number_of_teeth_cleaned < 10 then go back to step 3
- rinse toothbrush
The counter is called number_of_teeth_cleaned, and the condition is ‘if number_of_teeth_cleaned < 10’. This is very similar to selection as two routes are created. However, with iteration there is a loop back into the algorithm, rather than creating and keeping two separate routes as would occur with selection.
Now you’ve learnt the basic building blocks of an algorithm. In other articles we’ll dive deeper into the programming constructs that make up the algorithms in a software program and learn how to write elegant and effective code.
Interested in computer engineering? Find out more about all the computer engineering courses we have available by clicking here.
Diploma in Computer Engineering
Diploma in Artificial Intelligence
Alternatively, you can view all our online engineering courses here.
Recent Posts
What are the principles of operation of electrical cells?
What are the principles of operation of electrical cells? In our last article, we looked at the basic electrical parameters in D.C. circuits. In this article, we’re going to move on to the principles of operation of electrical cells. We’ll look at the different types of cells and batteries, their mechanisms, and their applications. What […]
How can we calculate basic electrical parameters?
How can we calculate basic electrical parameters? In our last article, we looked at how we can select the best engineering material for the task. In this article, we’re going to move on to basic electrical parameters in D.C. circuits. As an engineer, we need to be able to define these key terms and apply […]
How can we select engineering materials?
How can we select engineering materials? In our previous article, we looked at elastic and magnetic hysteresis. In this article, we’re going to look at how we can select engineering materials. In other articles, we have previously looked at the different materials and their properties. However, it’s important that engineers understand how we can go […]